JAVA was developed by James Gosling at Sun Microsystems Inc in the
year 1995, later acquired by Oracle Corporation. It is a simple
programming language. Java makes writing, compiling, and debugging
programming easy. It helps to create reusable code and modular
programs.
Java is a class-based, object-oriented programming language
and is designed to have as few implementation dependencies as
possible. A general-purpose programming language made for developers
to write once run anywhere that is compiled Java code can run on all
platforms that support Java. Java applications are compiled to byte
code that can run on any Java Virtual Machine. The syntax of Java is
similar to c/c++.
History
Java’s history is very interesting. It is a programming language
created in 1991. James Gosling, Mike Sheridan, and Patrick Naughton, a
team of Sun engineers known as the Green team initiated the Java
language in 1991. Sun Microsystems released its first public
implementation in 1996 as Java 1.0. It provides no-cost -run-times on
popular platforms. Java1.0 compiler was re-written in Java by Arthur
Van Hoff to strictly comply with its specifications. With the arrival
of Java 2, new versions had multiple configurations built for
different types of platforms.
In 1997, Sun Microsystems approached the
ISO standards body and later formalized Java, but it soon withdrew
from the process. At one time, Sun made most of its Java
implementations available without charge, despite their proprietary
software status. Sun generated revenue from Java through the selling
of licenses for specialized products such as the Java Enterprise
System.
On November 13, 2006, Sun released much of its Java virtual
machine as free, open-source software. On May 8, 2007, Sun finished
the process, making all of its JVM’s core code available under
open-source distribution terms.
The principles for creating java were
simple, robust, secured, high performance, portable, multi-threaded,
interpreted, dynamic, etc. In 1995 Java was developed by James
Gosling, who is known as the Father of Java. Currently, Java is used
in mobile devices, internet programming, games, e-business, etc.
Java programming language is named JAVA. Why?
After the name OAK, the team decided to give a new name to it and the
suggested words were Silk, Jolt, revolutionary, DNA, dynamic, etc.
These all names were easy to spell and fun to say, but they all wanted
the name to reflect the essence of technology. In accordance with
James Gosling, Java the among the top names along with Silk, and since
java was a unique name so most of them preferred it.
Java is the name of an island in Indonesia where the first
coffee(named java coffee) was produced. And this name was chosen by
James Gosling while having coffee near his office. Note that Java is
just a name, not an acronym.
Java Terminology
Before learning Java, one must be familiar with these
common terms of Java.
1. Java Virtual Machine(JVM): This is generally
referred to as JVM. There are three execution phases of a program.
They are written, compile and run the program.
- Writing a program is done by a java programmer like you and me.
- The compilation is done by the JAVAC compiler which is a primary Java compiler included in the Java development kit (JDK). It takes the Java program as input and generates bytecode as output.
- In the Running phase of a program, JVM executes the bytecode generated by the compiler.
Now, we understood that the function of Java Virtual Machine is to execute
the bytecode produced by the compiler. Every Operating System has a different
JVM but the output they produce after the execution of bytecode is the
same across all the operating systems. This is why Java is known as a
platform-independent language.
2. Bytecode in the Development process:
As discussed, the Javac compiler of JDK compiles the java source code
into bytecode so that it can be executed by JVM. It is saved as .class
file by the compiler. To view the bytecode, a disassembler like javap
can be used.
3. Java Development Kit(JDK): While we were using the
term JDK when we learn about bytecode and JVM. So, as the name
suggests, it is a complete Java development kit that includes
everything including compiler, Java Runtime Environment (JRE), java
debuggers, java docs, etc. For the program to execute in java, we need
to install JDK on our computer in order to create, compile and run the
java program.
4. Java Runtime Environment (JRE): JDK includes JRE. JRE
installation on our computers allows the java program to run, however,
we cannot compile it. JRE includes a browser, JVM, applet supports,
and plugins. For running the java program, a computer needs JRE.
5. Garbage Collector: In Java, programmers can’t delete the objects. To
delete or recollect that memory JVM has a program called Garbage
Collector. Garbage Collectors can recollect the objects that are not
referenced. So Java makes the life of a programmer easy by handling
memory management. However, programmers should be careful about their
code whether they are using objects that have been used for a long
time. Because Garbage cannot recover the memory of objects being
referenced.
6. ClassPath: The classpath is the file path where the
java runtime and Java compiler look for .class files to load. By
default, JDK provides many libraries. If you want to include external
libraries they should be added to the classpath.
Primary/Main Features of Java
1. Platform Independent: Compiler converts source code to
bytecode and then the JVM executes the bytecode generated by the
compiler. This bytecode can run on any platform be it Windows, Linux,
macOS which means if we compile a program on Windows, then we can run
it on Linux and vice versa. Each operating system has a different JVM,
but the output produced by all the OS is the same after the execution
of bytecode. That is why we call java a platform-independent language.
2. Object-Oriented Programming Language: Organizing the program in the
terms of collection of objects is a way of object-oriented
programming, each of which represents an instance of the class.
The four main concepts of Object-Oriented programming are:
- Abstraction
- Encapsulation
- Inheritance
- Polymorphism
3. Simple: Java is one of the
simple languages as it does not have complex features like pointers,
operator overloading, multiple inheritances, Explicit memory
allocation.
4. Robust: Java language is robust which means reliable.
It is developed in such a way that it puts a lot of effort into
checking errors as early as possible, that is why the java compiler is
able to detect even those errors that are not easy to detect by
another programming language. The main features of java that make it
robust are garbage collection, Exception Handling, and memory
allocation.
5. Secure: In java, we don’t have pointers, so we cannot
access out-of-bound arrays i.e it shows ArrayIndexOutOfBound Exception
if we try to do so. That’s why several security flaws like stack
corruption or buffer overflow are impossible to exploit in Java.
6. Distributed: We can create distributed applications using the java
programming language. Remote Method Invocation and Enterprise Java
Beans are used for creating distributed applications in java. The java
programs can be easily distributed on one or more systems that are
connected to each other through an internet connection.
7. Multithreading: Java supports multithreading. It is a Java feature
that allows concurrent execution of two or more parts of a program for
maximum utilization of CPU.
8. Portable: As we know, java code written
on one machine can be run on another machine. The platform-independent
feature of java in which its platform-independent bytecode can be
taken to any platform for execution makes java portable.
9. High Performance: Java architecture is defined in such a way that it
reduces overhead during the runtime and at some time java uses Just In
Time (JIT) compiler where the compiler compiles code on-demand basics
where it only compiles those methods that are called making
applications to execute faster.
10. Dynamic flexibility: Java being
completely object-oriented gives us the flexibility to add classes,
new methods to existing classes and even create new classes through
sub-classes. Java even supports functions written in other languages
such as C, C++ which are referred to as native methods.
11. Sandbox Execution: Java programs run in a separate space that allows user
to execute their applications without affecting the underlying system
with help of a bytecode verifier. Bytecode verifier also provides
additional security as its role is to check the code for any violation
of access.
12. Write Once Run Anywhere: As discussed above java
application generates a ‘.class’ file which corresponds to our
applications(program) but contains code in binary format. It provides
ease t architecture-neutral ease as bytecode is not dependent on any
machine architecture. It is the primary reason java is used in the
enterprising IT industry globally worldwide.
13. Power of compilation and interpretation: Most languages are designed
with purpose either they are compiled language or they are interpreted language.
But java integrates arising enormous power as Java compiler compiles the source
code to bytecode and JVM executes this bytecode to machine
OS-dependent executable code.
Example
// Demo Java program
// Importing classes from packages
import java.io.*;
// Main class
public class GFG{
// Main driver method
public static void main(String[] args){
// Print statement
System.out.println("Welcome to my freeCodeCamp.org project");
}
}
The Java language has undergone several changes since JDK 1.0 as well as numerous additions of classes and packages to the standard library. Since J2SE 1.4, the evolution of the Java language has been governed by the Java Community Process (JCP), which uses Java Specification Requests (JSRs) to propose and specify additions and changes to the Java platform. The language is specified by the Java Language Specification (JLS); changes to the JLS are managed under JSR 901. In September 2017, Mark Reinhold, chief Architect of the Java Platform, proposed to change the release train to "one feature release every six months" rather than the then-current two-year schedule. This proposal took effect for all following versions, and is still the current release schedule.
In addition to the language changes, other changes have been made to the Java Class Library over the years, which has grown from a few hundred classes in JDK 1.0 to over three thousand in J2SE 5. Entire new APIs, such as Swing and Java2D, have been introduced, and many of the original JDK 1.0 classes and methods have been deprecated. Some programs allow conversion of Java programs from one version of the Java platform to an older one (for example Java 5.0 backported to 1.4).
Regarding Oracle Java SE Support Roadmap, version 18 is that latest versions, and versions 17, 11 and 8 are the currently supported long-term support (LTS) versions, where Oracle Customers will receive Oracle Premier Support. Java 8 LTS last free software public update for commercial use was released by Oracle in January 2019, while Oracle continues to release no-cost public Java 8 updates for development and personal use indefinitely. Java 7 is no longer publicly supported. For Java 11, long-term support will not be provided by Oracle for the public; instead, the broader OpenJDK community, as Eclipse Adoptium or others, is expected to perform the work.
Java 18 General Availability began on March 22, 2022, and for Java 17, the latest (3rd) LTS on September 14, 2021. Java 19 is in development with early-access builds already available.
Variable in Java is a data container that saves the data values during Java program
execution. Every variable is assigned a data type that designates the type and quantity
of value it can hold. Variable is a memory location name of the data.
A variable is a name given to a memory location. It is the basic unit of storage in a
program.
- The value stored in a variable can be changed during program execution.
- A variable is only a name given to a memory location, all the operations done on the variable effects that memory location.
- In Java, all the variables must be declared before use.
How to declare variables?
We can declare variables in java as pictorially depicted below as a visual aid.

From the image, it can be easily perceived that while declaring a variable, we need to
take care of two things that are:
1. Datatype: Type of data that can be stored in this variable.
2. Dataname: Name was given to the variable.
In this way, a name can only be given to a memory location. It can be assigned values in two ways:
- Variable Initialization
- Assigning value by taking input
How to initialize variables?
It can be perceived with the help of 3 components that are as follows:
- datatype: Type of data that can be stored in this variable.
- variable_name: Name given to the variable.
- value: It is the initial value stored in the variable.

Types of Variables in Java
Now let us discuss different types of variables which are listed as follows:
- Local Variables
- Instance Variables
- Static Variables
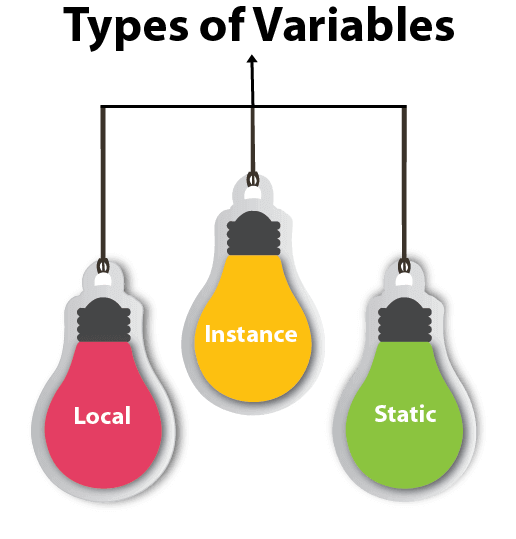
Let us discuss the traits of every variable been up here in detail.
1. Local Variables
A variable defined within a block or method or constructor is called a local variable.
- These variables are created when the block is entered, or the function is called and destroyed after exiting from the block or when the call returns from the function.
- The scope of these variables exists only within the block in which the variable is declared. i.e., we can access these variables only within that block.
- Initialization of the local variable is mandatory before using it in the defined scope.
import java.io.*;
class GFG {
public static void main(String[] args){
int var = 10; // Declared a Local Variable
// This variable is local to this main method only
System.out.println("Local Variable: " + var);
}
}
Output
Local Variable: 10
2. Instance Variables
Instance variables are non-static variables and are declared in a class outside any method, constructor, or block.
- As instance variables are declared in a class, these variables are created when an object of the class is created and destroyed when the object is destroyed.
- Unlike local variables, we may use access specifiers for instance variables. If we do not specify any access specifier, then the default access specifier will be used.
- Initialization of Instance Variable is not Mandatory. Its default value is 0
- Instance Variable can be accessed only by creating objects.
/*package whatever //do not write package name here */
import java.io.*;
class GFG {
public String geek; // Declared Instance Variable
public GFG() { // Default Constructor
this.geek = "Shubham Jain"; // initializing Instance Variable
}
//Main Method
public static void main(String[] args){
// Object Creation
GFG name = new GFG();
// Displaying O/P
System.out.println("Geek name is: " + name.geek);
}
}
Output
Geek name is: Shubham Jain
3. Static Variables
Static variables are also known as Class variables.
- These variables are declared similarly as instance variables. The difference is that static variables are declared using the static keyword within a class outside any method constructor or block.
- Unlike instance variables, we can only have one copy of a static variable per class irrespective of how many objects we create.
- Static variables are created at the start of program execution and destroyed automatically when execution ends.
- Initialization of Static Variable is not Mandatory. Its default value is 0
- If we access the static variable like the Instance variable (through an object), the compiler will show the warning message, which won’t halt the program. The compiler will replace the object name with the class name automatically.
- If we access the static variable without the class name, the compiler will automatically append the class name.
/*package whatever //do not write package name here */
import java.io.*;
class GFG {
public static String geek = "Shubham Jain";//Declared static variable
public static void main (String[] args) {
//geek variable can be accessed withod object creation
//Displaying O/P
//GFG.geek --> using the static variable
System.out.println("Geek Name is : "+GFG.geek);
}
}
Output
Geek Name is : Shubham Jain
Differences between the Instance variables and the Static variables
Now let us discuss the differences between the Instance variables and the Static variables:
- Each object will have its copy of the instance variable, whereas We can only have one copy of a static variable per class irrespective of how many objects we create.
- Changes made in an instance variable using one object will not be reflected in other objects as each object has its own copy of the instance variable. In the case of static, changes will be reflected in other objects as static variables are common to all objects of a class.
- We can access instance variables through object references, and Static Variables can be accessed directly using the class name.
Java Conditions and if Statements
Java supports the usual logical conditions from mathematics:
- Less than: a < b
- Less than or equal to: a <= b
- Greater than: a > b
- Greater than or equal to: a >= b
- Equal to a == b
- Not Equal to: a != b
You can use these conditions to perform different actions for different decisions.
Java has the following conditional statements:
- Use if to specify a block of code to be executed, if a specified condition is true
- Use else to specify a block of code to be executed, if the same condition is false
- Use else if to specify a new condition to test, if the first condition is false
- Use switch to specify many alternative blocks of code to be executed
The if Statement
Use the if statement to specify a block of Java code to be executed if a condition is true.
if (condition) {
// block of code to be executed if the condition is true
}
Note that if is in lowercase letters. Uppercase letters (If or IF) will generate an error.
In the example below, we test two values to find out if 20 is greater than 18. If the condition
is true, print some text:
if (20 > 18) {
System.out.println("20 is greater than 18");
}
We can also test variables:
int x = 20;
int y = 18;
if (x > y) {
System.out.println("x is greater than y");
}
The else Statement
Use the else statement to specify a block of code to be executed if the condition is false.
if (condition) {
// block of code to be executed if the condition is true
} else {
// block of code to be executed if the condition is false
}
Example
int time = 20;
if (time < 18) {
System.out.println("Good day.");
} else {
System.out.println("Good evening.");
}
// Outputs "Good evening."
The else if Statement
Use the else if statement to specify a new condition if the first condition is false.
if (condition1) {
// block of code to be executed if condition1 is true
} else if (condition2) {
// block of code to be executed if the condition1 is false and condition2 is true
} else {
// block of code to be executed if the condition1 is false and condition2 is false
}
Example
int time = 22;
if (time < 10) {
System.out.println("Good morning.");
} else if (time < 20) {
System.out.println("Good day.");
} else {
System.out.println("Good evening.");
}
// Outputs "Good evening."
Loops in Java
Looping in programming languages is a feature which facilitates the execution of a set of instructions/functions repeatedly while some condition evaluates to true. Java provides three ways for executing the loops. While all the ways provide similar basic functionality, they differ in their syntax and condition checking time.
- while loop: A while loop is a control flow statement that allows code to be
executed repeatedly based on a given Boolean condition. The while loop can be
thought of as a repeating if statement.
while (boolean condition){
loop statements...
}Flowchart:
- While loop starts with the checking of condition. If it evaluated to true, then the loop body statements are executed otherwise first statement following the loop is executed. For this reason it is also called Entry control loop.
- Once the condition is evaluated to true, the statements in the loop body are executed. Normally the statements contain an update value for the variable being processed for the next iteration.
- When the condition becomes false, the loop terminates which marks the end of its life cycle.
- for loop: for loop provides a concise way of writing the loop structure. Unlike a
while loop, a for statement consumes the initialization, condition and increment/decrement
in one line thereby providing a shorter, easy to debug structure of looping.
for (initialization condition; testing condition; increment/decrement){
statement(s)
}Flowchart:
- Initialization condition: Here, we initialize the variable in use. It marks the start of a for loop. An already declared variable can be used or a variable can be declared, local to loop only.
- Testing Condition: It is used for testing the exit condition for a loop. It must return a boolean value. It is also an Entry Control Loop as the condition is checked prior to the execution of the loop statements.
- Statement execution: Once the condition is evaluated to true, the statements in the loop body are executed.
- Increment/ Decrement: It is used for updating the variable for next iteration.
- Loop termination:When the condition becomes false, the loop terminates marking the end of its life cycle.
- do while: do while loop is similar to while loop with only difference that it checks
for condition after executing the statements, and therefore is an example of Exit Control Loop.
do{
statements...
}
while (condition);Flowchart:
- do while loop starts with the execution of the statement(s). There is no checking of any condition for the first time.
- After the execution of the statements, and update of the variable value, the condition is checked for true or false value. If it is evaluated to true, next iteration of loop starts.
- When the condition becomes false, the loop terminates which marks the end of its life cycle.
- It is important to note that the do-while loop will execute its statements atleast once before any condition is checked, and therefore is an example of exit control loop.
As the name suggests, Object-Oriented Programming or OOPs refers to languages that use
objects in programming. Object-oriented programming aims to implement real-world entities
like inheritance, hiding, polymorphism etc. in programming. The main aim of OOP is to bind
together the data and the functions that operate on them so that no other part of the code
can access this data except that function.
Let us discuss prerequisites by polishing concepts of method declaration and message passing.
Starting off with the method declaration, it consists of six components:
- Access Modifier: Defines the access type of the method i.e. from where it can be accessed
in your application. In Java, there are 4 types of access specifiers:
- public: Accessible in all classes in your application.
- protected: Accessible within the package in which it is defined and in its subclass(es) (including subclasses declared outside the package).
- private: Accessible only within the class in which it is defined.
- default (declared/defined without using any modifier): Accessible within the same class and package within which its class is defined.
- The return type: The data type of the value returned by the method or void if it does not return a value.
- Method Name: The rules for field names apply to method names as well, but the convention is a little different.
- Parameter list: Comma-separated list of the input parameters that are defined, preceded by their data type, within the enclosed parentheses. If there are no parameters, you must use empty parentheses ().
- Exception list: The exceptions you expect the method to throw. You can specify these exception(s).
- Method body: It is the block of code, enclosed between braces, that you need to execute to perform your intended operations.
Message Passing: Objects communicate with one another by sending and receiving information to
each other. A message for an object is a request for execution of a procedure and therefore
will invoke a function in the receiving object that generates the desired results. Message
passing involves specifying the name of the object, the name of the function and the information
to be sent.
Now that we have covered the basic prerequisites, we will move on to the 4 pillars of OOPs which
are as follows. But, let us start by learning about the different characteristics of an Object-Oriented
Programming Language.
OOPs concepts are as follows:
- Class
- Object
- Method and method passing
- Pillars of OOPs
- Abstraction
- Encapsulation
- Inheritance
- Polymorphism
- Compile-time polymorphism
- Runtime polymorphism
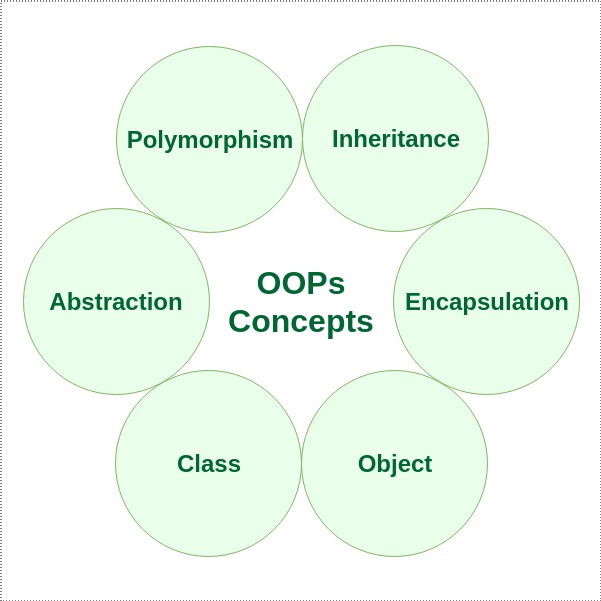
A class is a user defined blueprint or prototype from which objects are created. It represents the set of properties or methods that are common to all objects of one type. In general, class declarations can include these components in order:
- Modifiers: A class can be public or have default access (Refer this for details).
- Class name: The class name should begin with the initial letter capitalized by convention.
- Superclass (if any): The name of the class’s parent (superclass), if any, preceded by the keyword extends. A class can only extend (subclass) one parent.
- Interfaces (if any): A comma-separated list of interfaces implemented by the class, if any, preceded by the keyword implements. A class can implement more than one interface.
- Body: The class body surrounded by braces, { }.
Object is a basic unit of Object Oriented Programming that represents the real life entities. A typical Java program creates many objects, which as you know, interact by invoking methods. An object consists of:
- State: It is represented by the attributes of an object. It also reflects the properties of an object.
- Behavior: It is represented by the methods of an object. It also reflects the response of an object to other objects.
- Identity: It is a unique name given to an object that enables it to interact with other objects.
- Method: A method is a collection of statements that perform some specific task and return the result to the caller. A method can perform some specific task without returning anything. Methods allow us to reuse the code without retyping it, which is why they are considered time savers. In Java, every method must be part of some class, which is different from languages like C, C++ and Python.
Let us now discuss the 4 pillars of OOPs:
Pillar 1: Abstraction
Data Abstraction is the property by virtue of which only the essential details are displayed to the
user. The trivial or non-essential units are not displayed to the user. Ex: A car is viewed as a car
rather than its individual components.
Data Abstraction may also be defined as the process of identifying only the required characteristics
of an object, ignoring the irrelevant details. The properties and behaviors of an object
differentiate it from other objects of similar type and also help in classifying/grouping the
object.
Consider a real-life example of a man driving a car. The man only knows that pressing the
accelerators will increase the car speed or applying brakes will stop the car, but he does not know
how on pressing the accelerator, the speed is actually increasing. He does not know about the inner
mechanism of the car or the implementation of the accelerators, brakes etc. in the car. This is what
abstraction is.
In Java, abstraction is achieved by interfaces and abstract classes. We can achieve 100% abstraction
using interfaces.
Pillar 2: Encapsulation
It is defined as the wrapping up of data under a single unit. It is the mechanism that binds together the code and the data it manipulates. Another way to think about encapsulation is that it is a protective shield that prevents the data from being accessed by the code outside this shield.
- Technically, in encapsulation, the variables or the data in a class is hidden from any other class and can be accessed only through any member function of the class in which they are declared.
- In encapsulation, the data in a class is hidden from other classes, which is similar to what data-hiding does. So, the terms “encapsulation” and “data-hiding” are used interchangeably.
- Encapsulation can be achieved by declaring all the variables in a class as private and writing public methods in the class to set and get the values of the variables.
Pillar 3: Inheritance
Inheritance is an important pillar of OOP (Object Oriented Programming). It is the mechanism in
Java by which one class is allowed to inherit the features (fields and methods) of another class.
Let us discuss some frequently used important terminologies:
- Superclass: The class whose features are inherited is known as superclass (also known as base or parent class).
- Subclass: The class that inherits the other class is known as subclass (also known as derived or extended or child class). The subclass can add its own fields and methods in addition to the superclass fields and methods.
- Reusability: Inheritance supports the concept of “reusability”, i.e. when we want to create a new class and there is already a class that includes some of the code that we want, we can derive our new class from the existing class. By doing this, we are reusing the fields and methods of the existing class.
Pillar 4: Polymorphism
It refers to the ability of object-oriented programming languages to differentiate between entities with the same name efficiently. This is done by Java with the help of the signature and declaration of these entities.